In this post, we will learn how to check if a number is a perfect square in Python with a very simple example and algorithm, but before we jump into the programming part, let’s see what is a perfect square.
What is a Perfect Square?
A positive integer that is multiplied by itself and the resultant product is known as a perfect square.
For Example:
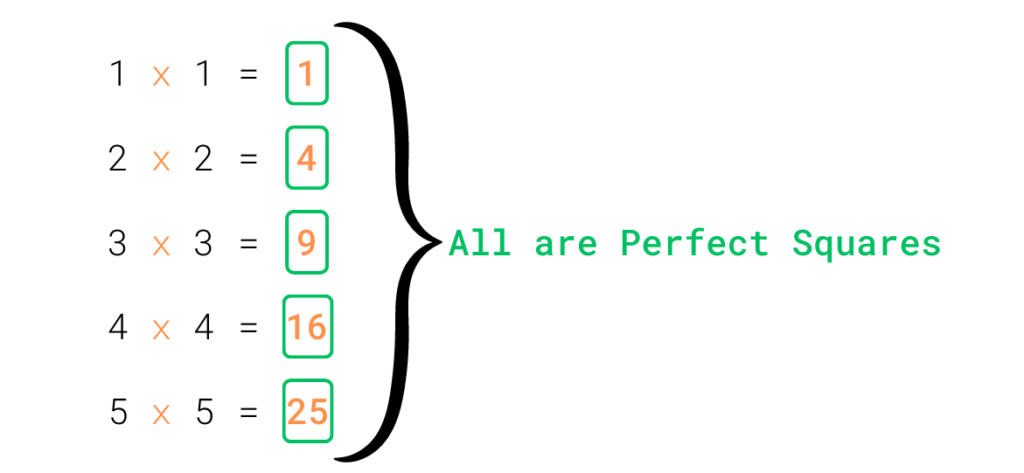
Algorithm for Perfect Square
- Take input from a user (
num
). - Create one variable called
flag
and initially set it to zero (flag = 0
). - iterate through the loop from 1 to num (
for i in range(1, num+1)
). - inside the loop check
if i*i == num
then do step-5 - increase the
flag
by 1 (flag = 1
) andbreak
the loop - Outside the loop check
if flag == 1
then print number is a perfect square. else
print number is not a perfect square
With the help of this algorithm, we will write the Python program to check if the number is a perfect square or not, but before writing this program few programming concepts you have to know:
Source Code
num = int(input("Enter a Number: "))
flag = 0
for i in range(1, num+1):
if i*i == num:
flag = 1
break
if flag == 1:
print(f"{num} is a Perfect Square")
else:
print(f"{num} is not a Perfect Square")
Output
Enter a Number: 9
9 is a Perfect Square
You can also use the sqrt()
function from the math
module to write this program. Let’s see how.
Python program to check if the number is a perfect square or not using the sqrt() function
Before writing this program you should know about:
- math module (
sqrt()
function) & - if-else
Source Code
import math
my_num = int(input("Enter A Number: "))
sq_root = int(math.sqrt(my_num))
if sq_root* sq_root == my_num:
print(f"{my_num} is a Perfect Square")
else:
print(f"{my_num} is not a Perfect Square")
Output
Enter A Number: 100
100 is a Perfect Square