In this post, you will learn what is a function in python, how to define a function, how to call a function, functions with parameters, default parameters, built-in functions, and so on.
So let’s start learning all the things one by one:
What is a function in Python?
A function is a block of code that performs a specific task when it is called, and functions are reusable, which means you can write a function once and use it as many times as you want.
Another definition:
A function is a block of code that you write once and can run as many times as you want in a program.
Types of functions
there are two types of functions in python and they are as follows.
- built-in function.
- user define function.
1. Built-in Function
Built-in Functions are predefined functions in python.
there are many built-in functions in python few are listed below.
List of built-in functions
print() | len() | min() |
max() | input() | type() |
dict() | list() | int() |
tuple() | set() | sorted() |
2. User define function
A function defined by the user is called the user-defined function and it performs its assigned task after calling it.
For Function two things are important.
- Function definition.
- Function call.
Define a function
def
keyword is used to define a function in python.
Example:
# define a function
def addtwo(a,b):
c = a+ b
return c
print(addtwo(3,5))
#calling a function
Output:
8
Function call
Calling a function is very easy just write a function name with a set of parenthesis.
example: addtwo(3,5)
(as shown in above program)
Below figure help you to understand all the terms related to function.
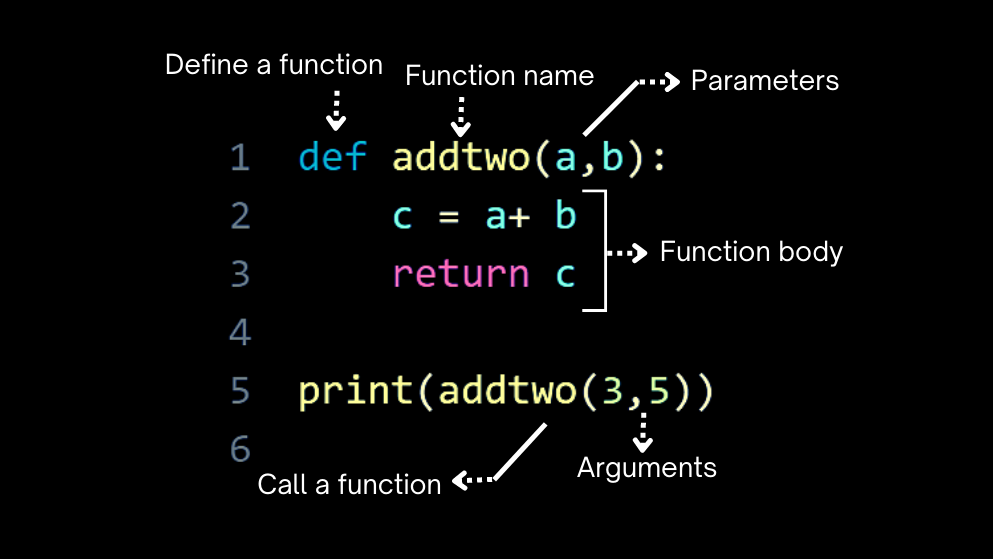
let’s do some programs using a function for better understanding.
Example for Practice
Write a Python program to check even and odd numbers by using a function.
Source code:
def odd_even(num):
if num % 2 == 0:
return 'Even'
else:
return 'Odd'
print(odd_even(3))
print(odd_even(4))
print(odd_even(6))
print(odd_even(5))
print(odd_even(7))
Output:
Odd
Even
Even
Odd
Odd
Write a python program to find the greatest number from three numbers
Source code:
def greatest(a,b,c):
if a>b and a>c:
return a
elif b>c:
return b
else:
return c
print(f"{greatest(45,35,53)} is greatest")
Output:
53 is greatest
Write a python program to check for palindrome.
Source code:
# check your name is same in reverse order or not
def name_check(n):
if n == n[::-1]:
return True
else:
return False
name = 'Saurabh'
name2 = 'naman'
print(f"{name} is palindrome :{name_check(name)}")
print(f"{name2} is palindrome : {name_check(name2)}")
Output:
Saurabh is palindrome :False
naman is palindrome : True
Call a function inside a function
Source code:
def greater(a,b):
if a>b:
return a
else:
return b
def greatest(a,b,c):
bigger = greater(a,b) # calling a greater() function here
return greater(bigger,c)
num1 = 75
num2 = 85
num3 = 35
print(f"{greatest(num1,num2,num3)} is greatest")
Output:
85 is greater
Default parameters
Below figure help you to understand, what are default parameters.
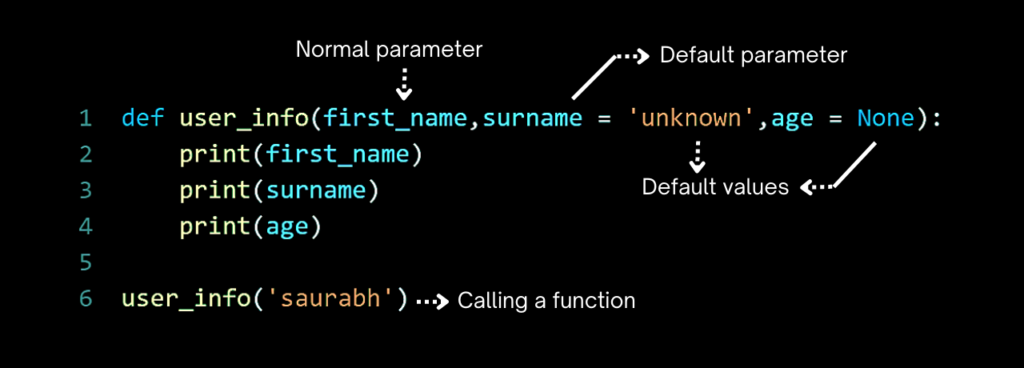
if values are not passed to default parameters then default values are printed and if values pass to default parameters then default values replace with passed values (or passed argument).
Example:
def default(name = 'unknown',surname = 'unknown',age = None):
print(f"your name is {name} ")
print(f"your surname is {surname} ")
print(f"your age is {age} ")
print('calling function with no arguments')
default()
# all default values printed because we are not pass any arguments
Output:
calling function with no arguments
your name is unknown
your surname is unknown
your age is None
When arguments pass in function then default parameters replace with passed arguments.
Example:
def default(name = 'unknown',surname = 'unknown',age = None):
print(name)
print(surname)
print(age)
default('saurabh','tiwari')
#default values replace with passed arguments
Output:
saurabh
tiwari
None
Here default values of name
& surname
variables replace with passed arguments.
NOTE: Always make the last parameter as a default parameter otherwise you will get an error (this thing is valid In case of using only default parameter and normal parameter at the same time).
Hope this post adds some value to your life -thank you.